MIDIイベントの処理
このチュートリアルでは、MIDI入力イベントの扱い方を説明します。外部ソースからMIDIデータを渡すことに加えて、オンスクリーンキーボードコンポーネントを紹介します。
レベル:中級
プラットフォーム:Windows, macOS, Linux
クラス: AudioDeviceManager,MidiMessage,MidiInputCallback,ComboBox,MidiKeyboardComponent,MidiKeyboardState,CallbackMessage,ScopedValueSetter
スタート
このチュートリアルのデモ・プロジェクトのダウンロードはこちらから:PIP|ZIP.プロジェクトを解凍し、最初のヘッダーファイルをProjucerで開く。
このステップにヘルプが必要な場合は、以下を参照してください。Tutorial: Projucer Part 1: Getting started with the Projucer.
コンピュータに外部MIDIソースを接続するのが理想的です。そうでない場合は、ある種のバーチャルMIDI音源(コンピューター上にバーチャルMIDIポートを作るもの)があると便利です。
デモ・プロジェクト
このデモ・プロジェクトでは、画面上にMIDIキーボードが表示され、コンボボックスを使ってハードウェア・デバイスのMIDI入力を1つ選択することができます。これらのソースから受信した MIDI イベントはウィンドウの下部に表示されます。これは次のスクリーンショットに示されています:
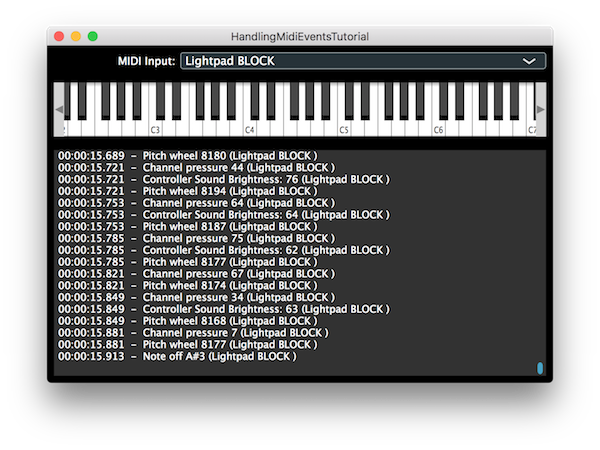
MIDI入力
このチュートリアルでは、基本的なアプリケーションで MIDI 入力を扱う方法を示します。JUCEは、接続されているハードウェアMIDIインタフェースのリストを簡単に見つけることができます。またMidiKeyboardComponentクラスのメンバ変数を見てみよう。まず、このクラス のメンバ変数を見てみよう。MainContentComponent
クラスである:
juce::AudioDeviceManager deviceManager; // [1]
juce::ComboBox midiInputList; // [2]
juce::Label midiInputListLabel;
int lastInputIndex = 0; // [3]
bool isAddingFromMidiInput = false; // [4]
juce::MidiKeyboardState keyboardState; // [5]
juce::MidiKeyboardComponent keyboardComponent; // [6]
juce::TextEditor midiMessagesBox;
double startTime;
- [1]を使用します。AudioDeviceManagerクラスを使用して、どのMIDI入力デバイスが有効になっているかを調べます。
- [2]MIDI入力デバイスの名前をこのコンボボックスに表示し、ユーザーが選択できるようにします。
- [3]別の入力を選択した際に、以前に選択したMIDI入力の登録を解除するために使用します。
- [4]このフラグは、オンスクリーンキーボードのマウスクリックではなく、外部ソースからMIDIデータが届いていることを示します。
- [5](その)MidiKeyboardStateクラスは、現在どのMIDI鍵盤が押されているかを記録します。
- [6]これはオンスクリーンキーボードコンポーネントです。
の中でMainContentComponent
コンストラクタで初期化します。[3],[4]そして[6].また、MIDIデータのタイムスタンプを相対的に表示できるように、アプリケーションの開始時間も記録します。
MainContentComponent()
: keyboardComponent (keyboardState, juce::MidiKeyboardComponent::horizontalKeyboard),
startTime (juce::Time::getMillisecondCounterHiRes() * 0.001)
{