アプリケーション・ウィンドウ
このチュートリアルでは、アプリケーション・ウィンドウを持つ最小限のアプリケーションを作成する方法と、そのウィンドウのサイズと外観をカスタマイズする方法を示します。これは、あらゆるJUCE GUIアプリケーションにとって重要です。
レベル初心者
プラットフォーム:Windows, macOS, Linux
クラス: JUCEApplication,DocumentWindow,ResizableWindow
スタート
Projucerを起動し、次の名前で新しいGUIアプリケーション・プロジェクトを作成します。メインウィンドウチュートリアル.での自動生成するファイル:フィールドでMain.cppファイルの みを作成する.
このステップでヘルプが必要な場合は、以下を参照してください。Tutorial: Projucer Part 1: Getting started with the Projucer.
を変更する。MainWindowTutorialApplication
クラスには以下が含まれる。MainWindow
クラスを以下のように変更した:
//==============================================================================
class MainWindowTutorialApplication : public juce::JUCEApplication
{
public:
//...
//==============================================================================
class MainWindow : public juce::DocumentWindow
{
public:
MainWindow (juce::String name) : DocumentWindow (name,
juce::Colours::lightgrey,
DocumentWindow::allButtons)
{
centreWithSize (300, 200);
setVisible (true);
}
void closeButtonPressed() override
{
juce::JUCEApplication::getInstance()->systemRequestedQuit();
}
private:
JUCE_DECLARE_NON_COPYABLE_WITH_LEAK_DETECTOR (MainWindow)
};
private:
std::unique_ptr mainWindow;
};
DocumentWindowA resizable window with a title bar and maximise, minimise and close buttons.Definition juce_DocumentWindow.h:67
nameint UnityEventModifiers const char * nameDefinition juce_UnityPluginInterface.h:204
JUCE_DECLARE_NON_COPYABLE_WITH_LEAK_DETECTOR#define JUCE_DECLARE_NON_COPYABLE_WITH_LEAK_DETECTOR(className)This is a shorthand way of writing both a JUCE_DECLARE_NON_COPYABLE and JUCE_LEAK_DETECTOR macro for ...Definition juce_PlatformDefs.h:262
ColoursContains a set of predefined named colours (mostly standard HTML colours)Definition juce_Colours.h:46
juceDefinition juce_AudioWorkgroup_mac.h:36
以下の行をinitialise()
関数である:
void initialise (const juce::String& commandLine) override
{
mainWindow.reset (new MainWindow (getApplicationName()));
}
最後に、以下の行をshutdown()
関数である:
void shutdown() override
{
mainWindow = nullptr;
}
デモ・プロジェクト
デモ・プロジェクトは、ソース・ファイルが1つしかない非常に最小限のJUCEアプリです:Main.cpp
.このアプリが行うのは、空のアプリケーション・ウィンドウを作成して表示することだけです。アプリを実行すると、このウィンドウが開くはずです:
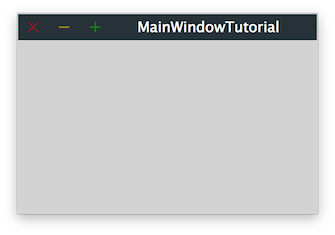
このウィンドウをドラッグして移動したり、最大化・最小化したり、閉じてJUCEアプリを終了することができます。
このアプリのC++コードは、動作するアプリに必要な最小限のコードに過ぎない。アプリケーション・クラスそのものはJUCEApplicationクラス)については、後のチュートリアルで取り上げる。今のところ、このアプリが実際に行っているのは、アプリのMainWindowTutorialApplication::initialise()
関数である:
void initialise (const juce::String& commandLine) override
{
mainWindow.reset (new MainWindow (getApplicationName()));
}
の新しいインスタンスを作成する。MainWindow
クラスで、アプリケーション・ウィンドウが表示される。
アプリケーション のinitialise()
関数が実行される。
メインウィンドウの実装
MainWindowクラス
では、この実装を見てみよう。MainWindow
クラスを継承している。これはJUCEDocumentWindowクラス、タイトル・バーのあるサイズ変更可能なウィンドウ、最大化、最小化、閉じるボタン。これらはすべて、アプリケーション・ウィンドウに期待される要素です。
一般的には、このようにしてアプリケーションのさまざまなコンポーネントを実装します。必要な機能を提供する適切なJUCE基本クラスを継承した新しいクラスを作成します。この新しいクラスでは、その上に独自のカスタム機能を追加することができます。
についてMainWindow
コンストラクタは以下のように定義されている:
MainWindow (juce::String name) : DocumentWindow (name,
juce::Colours::lightgrey,
DocumentWindow::allButtons)
{
centreWithSize (300, 200);
setVisible (true);
}
まず、ベースクラス(DocumentWindow)が初期化され、3つのパラメータを必要とする:
- タイトルバーに表示されるウィンドウの名前。
- ウィンドウの背景色。
- タイトルバーの隅にどのボタンを表示するか。
のコードを見てみよう。Main.cpp
最初のパラメーターは、実際にはMainWindow
アプリのinitialise()
関数。これは単にアプリの名前である(とThe Projucerプロジェクトが作成されたときに、アプリのバージョン番号と他のいくつかのものと一緒に)。
アプリのタイトルバーに表示される文字列を変更してみてください。
背景色の設定
次の2つの引数、背景色とボタンは、直接MainWindow
コンストラクタを使用します。ほとんどの一般的な色はJUCEで定義済みの定数を持っており、この例のように直接使用することができます。ですからColours::lightgrey定数Colours::redまたはColours::blueを使えば、アプリのウィンドウ全体の背景色を素早く変更できます。もちろん、独自のカスタムカラーを定義することも可能です。参照Tutorial: Colours in JUCEJUCEの色についてのより詳しい紹介はこちら。
ボタンにビットマスク引数を使う
に渡される第3引数。DocumentWindow基底クラスのコンストラクタはDocumentWindow::allButtons一定であることを示す。これは、デフォルトの3つのボタン(最大化、最小化、閉じる)をすべてタイトルバーに表示することを示します。このプロパティを変更するには、次の定数を使用します。enum
DocumentWindow::TitleBarButtons:
C++演算子の使用|
(ビット単位のOR演算子)を使えば、3つのボタンの組み合わせが定義できる。たとえば、閉じるボタンと最小化ボタンだけを表示するには、次のように入力します:
MainWindow (juce::String name) : DocumentWindow (name,
juce::Colours::lightgrey,
DocumentWindow::closeButton | DocumentWindow::minimiseButton)
{
centreWithSize (300, 200);
setVisible (true);
}
このように、フラグの組み合わせを関数に渡す方法をビットマスクJUCEの他の多くの場所で見られる。
ウィンドウサイズの設定
そのときMainWindow
オブジェクトが作成されると、コンストラクタの本体が実行されます。最初のステートメントでは、Component::centreWithSize()関数を呼び出してウィンドウの位置とサイズを設定します。2番目のステートメント、Component::setVisible()関数の呼び出しは、作成後にウィンドウを表示するために常に必要です。
Component::centreWithSize() 関数を使用すると、ウィンドウの最初の幅と高さをピクセル単位で指定できます。この関数は、指定されたサイズを設定し、親コンポーネントに対してコンポーネントをセンタリングします。この関数はDocumentWindowクラスはTopLevelWindowクラスは親コンポーネントを持たないので、画面全体に対して中央に配置される。
ウィンドウの別の位置を指定したい場合もあるでしょ う。この場合、Component::setBounds()関数を使用すると、より多くのオプションを指定できます。これを試すには、関数centreWithSize()
関数をこれに追加する:
setBounds (50, 50, 800, 600);
これにより、サイズが800×600ピクセルに設定され、ウィンドウの位置が画面左上に近づきます(画面端から50ピクセル離れます)。
Component::setBoundsRelative()関数を使用して、絶対ピクセルではなく、画面サイズからの相対的な位置とサイズを設定します。
サイズ、バウンズ、ポジションについては、以下をご覧ください。Tutorial: Parent and child components.
その他のカスタマイズ・オプション
ウィンドウをリサイズ可能にする
JUCEDocumentWindowクラス自体はResizableWindowクラスで定義されています。この基底クラスは、ユーザがウィンドウのサイズを変更できるようにする機能を追加します。これは関数ResizableWindow::setResizable().
以下の行をMainWindow
ビルダー
setResizable (true, true);
のドキュメントを見てみよう。ResizableWindow::setResizable()関数の2つのブーリアン引数の意味を調べる。最初の引数はウィンドウのサイズを変更できるかどうかを決定します。番目の引数は、ウィンドウのサイズを変更するために右下にハンドルを追加するかどうかを決定します。この引数がfalse
ウィンドウの端をマウスでドラッグすると、ウィンドウのサイズを変更できる。
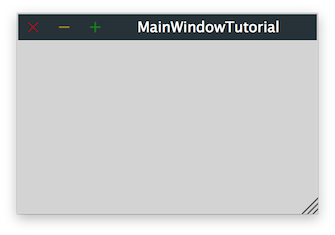
ネイティブタイトルバーの使用
これまでのところ、アプリのメイン・ウィンドウにはJUCEのルック&フィールを使っている。その主な利点は、どのデスクトップ・オペレーティング・システム(Windows、Mac OS X、Linux)でも同じように見え、同じように動作することです。
しかし、JUCEはDocumentWindowクラスを使用すると、ウィンドウを実行するオペレーティング・システムの典型的なネイティブ・ウィンドウの外観を使用できるようになります。これは関数DocumentWindow::setUsingNativeTitleBar().以下の行をMainWindow
ビルダー
setUsingNativeTitleBar (true);
これでアプリケーションのウィンドウはこのようになる:
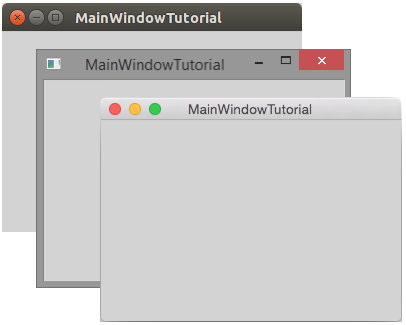
これにより、ウィンドウの外観だけでなく、動作もネイティブ・オペレーティング・システムのウィンドウと同じように調整されることに注意してください。例えばuseBottomRightCornerResizer
引数をResizableWindow::setResizable()関数はウィンドウのリサイズ動作を変更しません。その代わりに、マウスによるサイズ変更では、ウィンドウは実行中のオペレーティング・システムのネイティブなルック・アンド・フィールを採用します。
ほとんどのアプリの場合、メインアプリのウィンドウはネイティブのルック&フィールの方が適しています。
より多くのカスタマイズ・オプション
のドキュメントをご覧ください。DocumentWindowそして